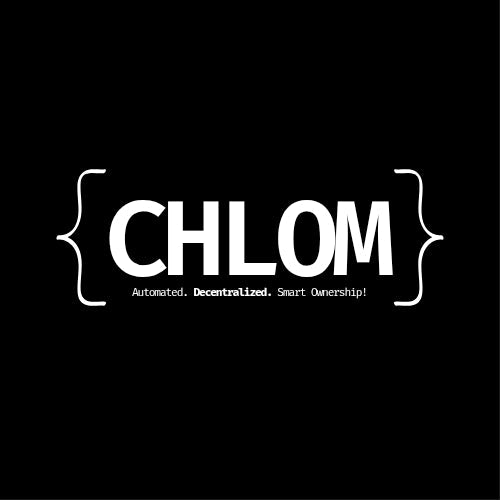
CHLOM™ Technical Whitepaper for Builders
Share
CHLOM™ provides a decentralized framework for licensing, compliance, and revenue distribution through blockchain-powered smart contracts and AI-driven automation. This whitepaper outlines how developers can implement CHLOM™ APIs, SDKs, and smart contracts to integrate CHLOM™ into their platforms.
🔹 CHLOM™ System Overview
The CHLOM™ ecosystem consists of modular components:
- CHLOM™ API – Provides REST and Web3 endpoints for licensing, compliance, and financial management.
- Smart Contracts – Solidity-based self-executing contracts governing licensing terms.
- CHLOM™ SDK – A set of development tools for integrating licensing automation in applications.
- AI-Driven Compliance Engine – Automates auditing, enforcement, and contract validation.
- CHLOM™ Token ($CHLOM) – Used for staking, governance, and transaction execution.
🔹 CHLOM™ API: REST & Web3 Endpoints
1. Authentication & License Verification
All API requests must be authenticated via API keys or Web3 signatures.
# Example: Fetch license details via REST API GET /api/v1/license/{license_id} Headers: Authorization: Bearer {API_KEY} Response: { "license_id": "0x123...", "owner": "0xabc...", "status": "active", "expiration": "2026-01-01" }
# Example: Web3 authentication using Ethereum Wallet async function authenticateUser(walletAddress, signature) { const response = await fetch('/api/v1/authenticate', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ walletAddress, signature }) }); return response.json(); }
2. Smart Contract Interaction via API
# Example: Execute a licensing smart contract POST /api/v1/smart-contract/execute Body: { "contract_address": "0x123...", "function_name": "registerLicense", "parameters": ["0xabc...", "enterprise_license"] }
🔹 CHLOM™ SDK: Developer Integration Toolkit
Installation
# Install CHLOM SDK via npm npm install chlom-sdk
Usage Example
// Import the SDK const ChlomSDK = require('chlom-sdk'); // Initialize SDK const chlom = new ChlomSDK({ apiKey: "your_api_key" }); // Fetch license details async function getLicenseDetails(licenseId) { const license = await chlom.getLicense(licenseId); console.log(license); }
🔹 CHLOM™ Smart Contracts (Solidity)
License Management Smart Contract
pragma solidity ^0.8.0; contract ChlomLicense { address public owner; mapping(address => bool) public licenseHolders; event LicenseIssued(address indexed holder); constructor() { owner = msg.sender; } function issueLicense(address _holder) public { require(msg.sender == owner, "Only owner can issue licenses"); licenseHolders[_holder] = true; emit LicenseIssued(_holder); } function verifyLicense(address _holder) public view returns (bool) { return licenseHolders[_holder]; } }
Revenue Sharing Smart Contract
pragma solidity ^0.8.0; contract RevenueSharing { address public owner; mapping(address => uint256) public balances; event PaymentReceived(address indexed payer, uint256 amount); event PaymentDistributed(address indexed receiver, uint256 amount); constructor() { owner = msg.sender; } function deposit() public payable { require(msg.value > 0, "Must send Ether"); balances[msg.sender] += msg.value; emit PaymentReceived(msg.sender, msg.value); } function distribute(address payable receiver, uint256 amount) public { require(msg.sender == owner, "Only owner can distribute funds"); require(address(this).balance >= amount, "Insufficient balance"); receiver.transfer(amount); emit PaymentDistributed(receiver, amount); } }
🔹 AI-Driven Compliance & Automation
1. Automated Licensing Audits
import openai def analyze_license_compliance(license_data): response = openai.Completion.create( model="gpt-4", prompt=f"Analyze this licensing data: {license_data}", max_tokens=50 ) return response["choices"][0]["text"]
2. Smart Contract Auditing
# Example: AI-powered smart contract audit POST /api/v1/ai/audit-smart-contract Body: { "contract_address": "0x123...", "audit_type": "security" }
🔹 Integration Strategy & Deployment
1. Local Development
- Run CHLOM API in a local Docker container.
- Deploy smart contracts on a testnet (e.g., Ethereum Goerli).
- Test SDK integration with real-time license validation.
2. Production Deployment
- Deploy CHLOM API and smart contracts on Ethereum mainnet.
- Enable on-chain governance mechanisms.
- Establish liquidity staking pools for $CHLOM tokens.
🔹 Future Enhancements
- Integration with Layer 2 scaling solutions.
- Cross-chain interoperability with Solana, Polygon, and Avalanche.
- Expansion of AI compliance tools for real-time monitoring.
🔹 Conclusion
CHLOM™ provides a scalable, automated licensing framework that enables secure transactions, decentralized governance, and compliance enforcement. By leveraging smart contracts, AI-driven monitoring, and modular SDKs, developers can seamlessly integrate CHLOM™ into their platforms.
Next Steps: Developers should start by:
- Setting up CHLOM™ API & SDK.
- Deploying smart contracts in a test environment.
- Exploring staking and governance integrations.
For full documentation, visit our developer portal.