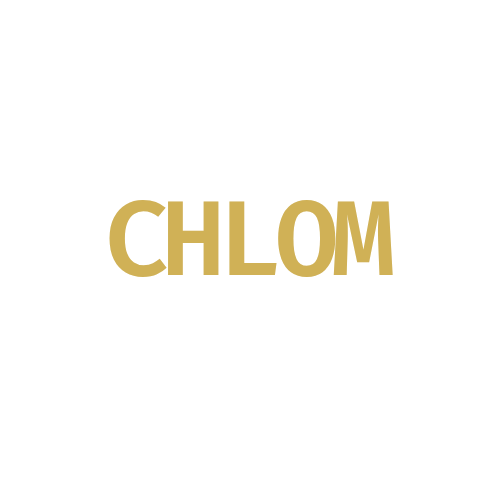
CHLOM™ Blockchain
Share
AI-Powered Decentralized Licensing, Compliance, and Smart Governance
Version: 1.0 | Last Updated: February 2025
1. Introduction
The CHLOM™ Blockchain is an advanced decentralized framework integrating AI-driven compliance, decentralized governance, tokenized licensing, smart treasury management, and Zero-Knowledge Proof (ZKP) authentication. This blockchain-based infrastructure enables automated, trustless licensing and royalty distribution while ensuring security, efficiency, and interoperability across multiple industries.
By leveraging a hybrid Proof-of-Stake (PoS) and Proof-of-Authority (PoA) consensus mechanism, CHLOM™ ensures scalability and low-cost transactions, making it ideal for licensing, governance, and decentralized compliance automation.
2. Core Components of CHLOM™ Blockchain
- Hybrid PoS/PoA Consensus: Secure, scalable validation mechanism for decentralized compliance.
- CHLOM Token Standard: Dual-token system enabling on-chain licensing and utility functions.
- License Exchange (LEX): Decentralized licensing marketplace with royalty automation.
- Smart Treasury: Automated tax collection and royalty distribution.
- Decentralized Governance (DAO): AI-powered, community-driven policy enforcement.
- ZKP Authentication: Privacy-preserving identity verification for compliance.
- Cross-Chain Interoperability: Bridge for multi-chain licensing and smart contract interaction.
3. Blockchain Consensus & Network Layer
3.1 CHLOM Blockchain Node (Go)
The foundational node client, written in Go, maintains the blockchain state, executes consensus, and manages peer-to-peer transactions.
package main import ( "fmt" "net" "encoding/json" "time" "sync" ) type Block struct { Index int Timestamp string PrevHash string Hash string Data string Validator string } type Node struct { Address string Peers []string Chain []Block mux sync.Mutex } func NewBlock(prev Block, data string, validator string) Block { return Block{ Index: prev.Index + 1, Timestamp: time.Now().String(), PrevHash: prev.Hash, Hash: fmt.Sprintf("%x", time.Now().UnixNano()), Data: data, Validator: validator, } } func (node *Node) AddBlock(data string, validator string) { node.mux.Lock() defer node.mux.Unlock() newBlock := NewBlock(node.Chain[len(node.Chain)-1], data, validator) node.Chain = append(node.Chain, newBlock) fmt.Println("Block Added:", newBlock) } func main() { genesisBlock := Block{Index: 0, Timestamp: time.Now().String(), PrevHash: "", Hash: "genesis", Data: "Genesis Block", Validator: "CHLOM DAO"} node := Node{Address: "127.0.0.1:8080", Chain: []Block{genesisBlock}} select {} }
4. Smart Contracts & Licensing Infrastructure
4.1 CHLOM Token Standard (Solidity)
The CHLOM Token powers licensing transactions, TLaaS (Tokenized Licensing-as-a-Service), and decentralized finance within the ecosystem.
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; import "@openzeppelin/contracts/token/ERC20/ERC20.sol"; import "@openzeppelin/contracts/access/Ownable.sol"; contract CHLOMToken is ERC20, Ownable { constructor() ERC20("CHLOM Coin", "CHLOM") { _mint(msg.sender, 1000000000 * 10 ** decimals()); } function mint(address to, uint256 amount) public onlyOwner { _mint(to, amount); } function burn(uint256 amount) public { _burn(msg.sender, amount); } }
4.2 Decentralized License Exchange (LEX)
LEX enables the minting, transfer, and sublicensing of digital and real-world licenses.
pragma solidity ^0.8.0; contract LicenseExchange { struct License { uint256 id; address owner; uint256 price; string metadata; bool transferable; } mapping(uint256 => License) public licenses; uint256 public nextLicenseId = 1; function mintLicense(string memory metadata, uint256 price, bool transferable) public { licenses[nextLicenseId] = License(nextLicenseId, msg.sender, price, metadata, transferable); nextLicenseId++; } function transferLicense(uint256 id, address to) public { require(licenses[id].transferable, "License is non-transferable"); require(licenses[id].owner == msg.sender, "Not owner"); licenses[id].owner = to; } }
5. AI-Driven Compliance & Fraud Detection
5.1 AI Model for Fraud Detection (Python)
import numpy as np import joblib from sklearn.ensemble import RandomForestClassifier from sklearn.preprocessing import StandardScaler class CHLOMFraudDetection: def __init__(self): self.model = RandomForestClassifier(n_estimators=100) self.scaler = StandardScaler() def train(self, X, y): X_scaled = self.scaler.fit_transform(X) self.model.fit(X_scaled, y) joblib.dump(self.model, "fraud_model.pkl") def predict(self, transaction_data): X_scaled = self.scaler.transform([transaction_data]) return self.model.predict(X_scaled)
6. Zero-Knowledge Proof (ZKP) Authentication
6.1 Secure ZKP-Based Identity Verification
import py_ecc.bn128 as bn128 class CHLOMZKP: def __init__(self, secret): self.secret = secret def generate_proof(self): return bn128.multiply(bn128.G1, self.secret) def verify_proof(self, proof): return bn128.pairing(proof, bn128.G2)
7. CHLOM DAO: Governance & Treasury
7.1 CHLOM DAO Smart Contract
pragma solidity ^0.8.0; contract CHLOMDAO { struct Proposal { uint256 id; string description; uint256 voteCount; bool executed; } mapping(uint256 => Proposal) public proposals; uint256 public nextProposalId = 1; function createProposal(string memory description) public { proposals[nextProposalId] = Proposal(nextProposalId, description, 0, false); nextProposalId++; } function vote(uint256 proposalId) public { proposals[proposalId].voteCount++; } function executeProposal(uint256 proposalId) public { require(proposals[proposalId].voteCount > 100, "Not enough votes"); proposals[proposalId].executed = true; } }
8. Conclusion
The CHLOM™ Blockchain establishes a decentralized ecosystem where licensing, compliance, governance, and smart treasury management are executed in a trustless, AI-powered framework. By integrating TLaaS, ZKP authentication, and AI-driven risk assessment, CHLOM™ enables seamless automation of licensing and governance across industries.
As builders integrate components, XENthrive will transition governance and assets to the CHLOM DAO, ensuring sustainability, decentralization, and self-regulation.